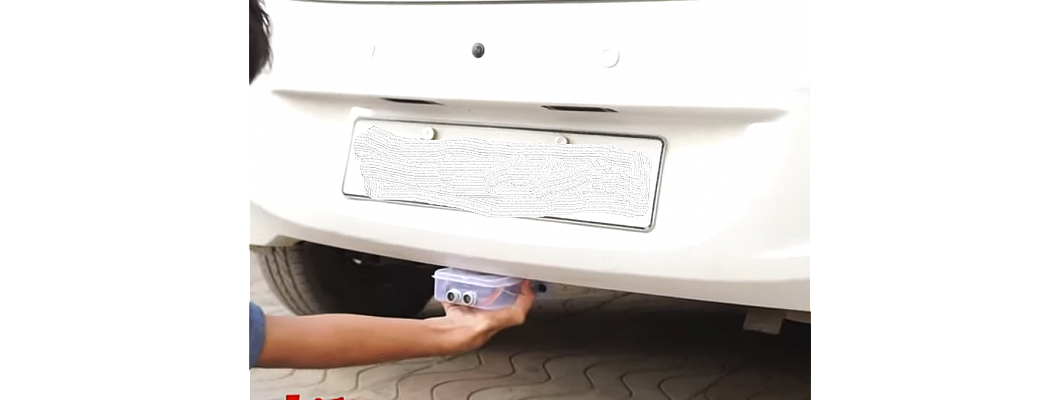
Hello,
In this tutorial, we are going to learn how to make a reverse parking alert system at home using Arduino.
For this project, we can use various sensors like infrared sensors, proximity sensors, etc. But we are going to use the ultrasonic HC-SR04 sonar module as it is very compact and gives a great performance.
Hardware Required
Software Required
Circuit diagram
-
The circuit for this project consists of 4 different components: The Buzzer, The Ultrasonic sensor, the Arduino Nano, and the power supply.
Pins on the ultrasonic sensor |
Pins on the Arduino |
VCC |
5V |
GND |
GND |
TRIG |
D12 |
ECHO |
D11 |
Pins on the Buzzer |
Pins on the Arduino |
Positive terminal |
5V |
Negative terminal |
GND |
Signal pin |
D8 |
-
The positive and negative terminals of the battery are connected to the VIN and GND pins of the Arduino Nano.
Assembly and Working concept
-
Make holes in the case for the ultrasonic sensor. Fix the ultrasonic sensor into the case.
-
Now connect the Buzzer and the ultrasonic sensor to the Arduino board.
-
Finally, connect the power supply to the Arduino board.
-
Fit everything inside the case. Stick the case to the back of the vehicle like shown in the picture.
-
The working concept of this project consists of three different processes.
-
Firstly, as we know the ultrasonic sensor works on the principle of Sonar, so the transmitter emits the ultrasonic waves and the detector detects the waves.
-
In case of any obstacles, the ultrasonic waves will reflect the sensor, and the detector will detect that there is an object and will calculate the distance and send it to the Arduino board.
-
The Arduino board will further send the command to the buzzer to make noise so the driver can understand that there is an obstacle nearby.
-
Furthermore, the code is designed in such a way that the buzzer will buzz with a higher frequency as the distance of the obstacle is less.
Arduino Code
- The Arduino code for this project is mentioned below.
- Upload the code.
#define trigPin 12 #define echoPin 11 int Buzzer = 8; int duration, distance; void setup() { Serial.begin (9600); pinMode(trigPin, OUTPUT); pinMode(echoPin, INPUT); pinMode(Buzzer, OUTPUT); } void loop() { digitalWrite(trigPin, HIGH); delayMicroseconds(1000); digitalWrite(trigPin, LOW); duration = pulseIn(echoPin, HIGH); distance = (duration / 2) / 29.1; if (distance <= 100 && distance >= 70) { Serial.println("object detected \n"); Serial.print("distance= "); analogWrite(Buzzer, 0); delay (500) ; analogWrite(Buzzer, 255); delay (5000) ; } else if (distance <= 80 && distance >= 50) { Serial.println("object detected \n"); Serial.print("distance= "); analogWrite(Buzzer, 0); delay (450) ; analogWrite(Buzzer, 255); delay (450) ; } else if (distance <= 50 && distance >= 30) { Serial.println("object detected \n"); Serial.print("distance= "); analogWrite(Buzzer, 0); delay (250) ; analogWrite(Buzzer, 255); delay (250) ; } else if (distance <= 30 && distance >= 20) { Serial.println("object detected \n"); Serial.print("distance= "); analogWrite(Buzzer, 0); delay (150) ; analogWrite(Buzzer, 255); delay (150) ; } else if (distance <= 20 && distance >= 10) { Serial.println("object detected \n"); Serial.print("distance= "); analogWrite(Buzzer, 0); delay (100) ; analogWrite(Buzzer, 255); delay (100) ; } else if (distance <= 10 && distance > 5) { Serial.println("object detected \n"); Serial.print("distance= "); analogWrite(Buzzer, 0); delay (50) ; analogWrite(Buzzer, 255); delay (50) ; } else if (distance <= 5 && distance >= 1) { Serial.println("object detected \n"); Serial.print("distance= "); analogWrite(Buzzer, 0); delay (10) ; analogWrite(Buzzer, 255); delay (10) ; } else Serial.println("object detected \n"); Serial.print("distance= "); Serial.print(distance); analogWrite(Buzzer, 255); { } }
Leave a Comment