15 Jul
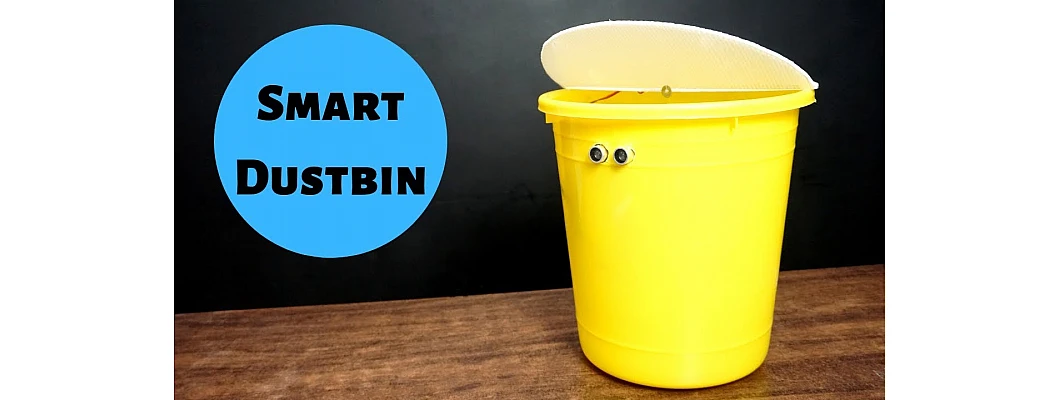
Hello,
In this tutorial based article, we are going to learn how to make a smart dustbin using Arduino Uno and an ultrasonic sensor.
Hardware Required
Software Required
Arduino Uno
- Arduino Uno is an open-source microcontroller board based on the processor ATmega328P. It has 14 digital input/output pins (of which 6 can be used as PWM outputs), 6 analog inputs, a USB connection, a power jack, an ICSP header and a reset button. It contains everything needed to support the microcontroller. Just plug it into a computer with a USB cable or power it with an adapter to get started. You can experiment with your Arduino without worrying too much about it. In the event of a worst case scenario, you could buy a new one as the Uno is very economical
Ultrasonic Sensor HC-SR04
- HC-SR04 is an ultrasonic distance sensor used for measuring the distance at which an object is located. The principle used by this sensor is called SONAR. It is perfect for small robotics projects such as obstacle avoiding robot, distance measuring device etc. It has two parts, one emits the ultrasound sonar to measure the distance to an object. The other part is the receiver which listens for the echo. As soon as the ultrasound hits the object it bounces back and is detected by the receiver. The time taken for the wave to come back decides the distance of the object being measured.
SG90 Micro servo motor
- The micro servo 9G is a light, good quality and very fast servo motor. This servo is designed to work with most radio control systems. It is perfect for small robotics projects. The SG90 mini servo with accessories is perfect for remote-controlled helicopters, planes, cars, boat,s and trucks.
Working Concept
- It is important to dispose of the trash properly. It is a responsibility with which everyone should comply. In the era of Covid-19, people are trying to innovate everyday life things and make things as contactless as possible. Smart dustbin is one of those innovative ideas.
- The smart dustbin uses an Ultrasonic sensor HC-SR04 to detect objects in front.
- It then sends the signals to Arduino Uno. The Arduino understands the signal and sends a signal to the Servomotor which opens the flap on top of the dustbin.
- Here we have program it to open the race for only 3 seconds after 3 seconds the flap automatically closes. You can change that time just by making minor changes to the code in Arduino IDE.
- The work is shown below.
Circuit Diagram
- The circuit diagram for smart dustbin contains three main components: Arduino Uno, Power supply and an ultrasonic sensor.
- The Ultrasonic sensor HC-SR04 pins echo and trig are connected to Arduino Uno pins 5 and 6 respectively. The VCC pin is connected to 5V on Arduino Uno and both the grounds are connected together.
-
Servo motor signal pin to 7 and gnd to gnd and positive to 3.3 V
- A 9v battery has been connected to Vin pin on the Arduino Uno and grounds are connected together.
Program code
The program for the Arduino code can be downloaded here. Upload the code to Arduino Uno. Stick the Arduino, and battery on the wall of the dustbin using a double-sided tape.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
#include |
Now your smart dustbin is ready for use! Enjoy!
Leave a Comment