09 Feb
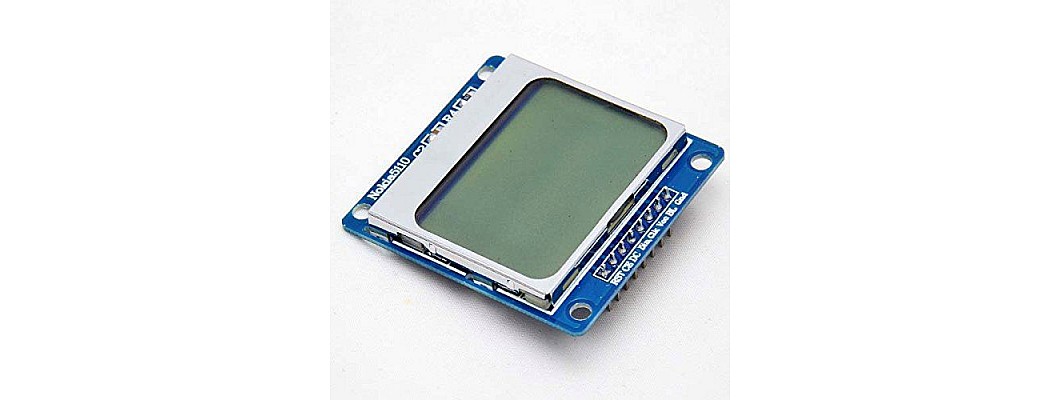
Hello,
In this tutorial, we are going to learn about the Nokia 5110 display and its interfacing with Arduino.
In this tutorial, we are going to learn about the Nokia 5110 display and its interfacing with Arduino.
Hardware Required
Software Required
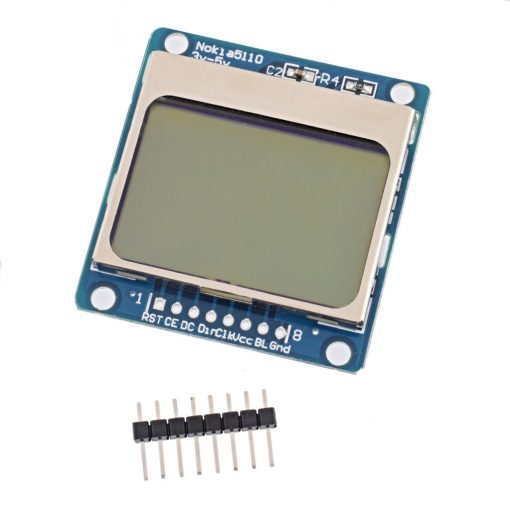
- The Nokia 5110 LCD is a very versatile display and can be used to present any text or Bitmap image. It has an interior controller/Driver PCD8544. With this driver, the data is converted and displayed onto the display. PCD8544 is a low power controller which is designed to drive a graphic display of 48 rows and 84 columns.
- All the functions required by the display are done through this single chip. This includes the bias voltages and on-chip generation of LCD supply. This leads to small size as there are no external components and a low power consumption.
- The PCD8544 directly interfaces with microcontrollers using a serial bus. The PCD8544 is manufactured using the CMOS technology.
- Nokia 5110 display uses the SPI protocol for interfacing with microcontrollers and which might even be called MOSI (Master In Slave Out). So, all we will do is pass commands in 8-bit data through the MOSI pin.
Specifications
- 48 X 84 Dot LCD
- SPI protocol with maximum high speed 4.0 Mbits/S
- PCD8544 driver
- LED Backlit
- Run at Voltage 2.7-5 volt
- Low power consumption
- Support signal CMOS input
Pinout
- RST - RESET pin for the operation of LCD.
- CE - Enable chip pin.
- D/C - Pin to configure the formats between Data and Command.
- DIN - Serial Input pin for data transfer.
- CLK - Serial clock pin.
- VCC - 3.3V power supply pin from microcontroller.
- LIGHT - Backlight control.
- GND - Ground pin.
Interfacing with Arduino
Nokia 5110 LCD
|
Arduino Uno
|
RST
|
3
|
CE
|
4
|
DC
|
5
|
DIN
|
6
|
CLK
|
7
|
VCC
|
3.3V
|
BL
|
3.3V
|
GND
|
GND
|
Arduino Code
- Download the PCD8544 Nokia and the Adafruit GFX library from the manage libraries section in the Arduino IDE.
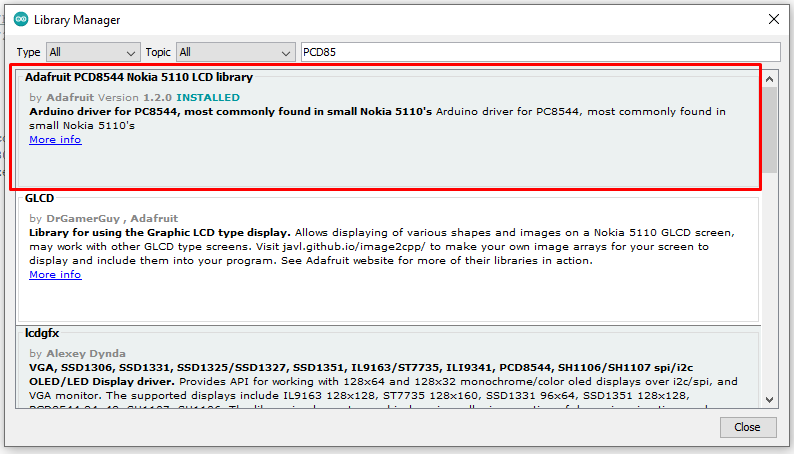
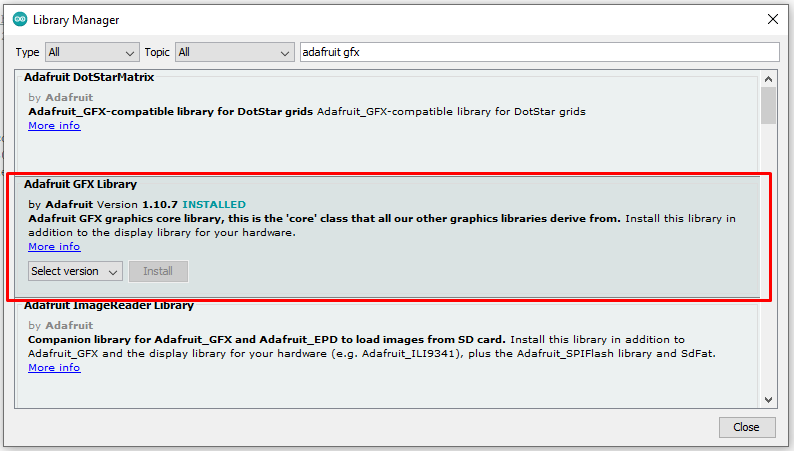
- Upload the code to the Arduino board.
#include <SPI.h> #include <Adafruit_GFX.h> #include <Adafruit_PCD8544.h> Adafruit_PCD8544 display = Adafruit_PCD8544(7, 6, 5, 4, 3); void setup() { display.begin(); display.setContrast(57); display.clearDisplay(); display.setTextColor(WHITE, BLACK); display.setCursor(4,8); display.setTextSize(1); display.println("|Electronics|"); display.setCursor(28,20); display.println("|Hub|"); display.setCursor(12,32); display.setTextColor(BLACK); display.println("Nokia 5110"); display.display(); delay(1000); } void loop() { }
Leave a Comment