06 Feb
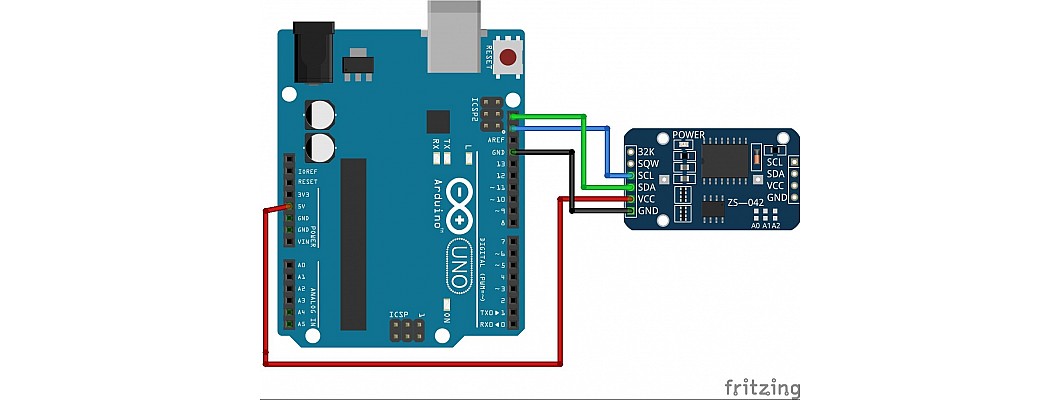
Hello,
In this tutorial, we are going to build a clock with LCD Display and DS3231 RTC module.
In this tutorial, we are going to build a clock with LCD Display and DS3231 RTC module.
Hardware Required
Software Required
- Arduino IDE
- Alarm clocks are one of the most common electronic items in every household. But first let us talk about the heart of this project which is the DS3231 RTC module.
- The central processor of this RTC module is a very cheap, extremely powerful and accurate DS3231 Chip by Maxim.
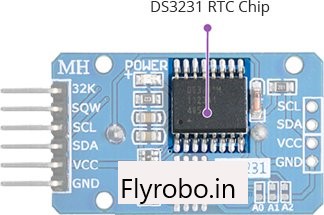
- The chip keeps track of seconds, minutes, hours, days, months and year's information. The number of days in a month are adjusted by the chip accordingly be it 30, 31 or 28. It can keep track of all this till the year 2100.
- It can keep both 24-hour or 12-hour information with AM/PM settings. It can also store up to two alarms.
- Almost all RTC modules come with a 32kHz crystal which helps in the process of time-keeping. The main issue with this crystal is that the change in surrounding temperatures can affect the frequency of these crystals. These changes are negligible in the short term but will affect the time keeping process in the long term.
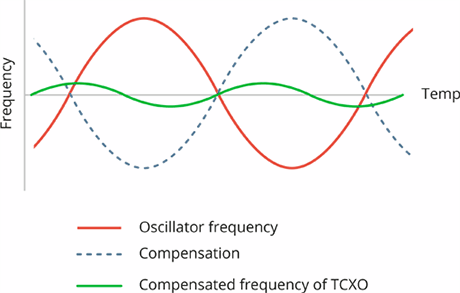
- To avoid such drifts, the DS3231 module uses a temperature compensated crystal oscillator (TCXO). This generates a frequency reverse to that of the oscillator frequency to compensate the changes and thus keeps the frequency of the crystal unchanged.
- TCXO is the reason that the RTC module can maintain an accuracy of 2 minutes per year.
- The DS3231 module is run using battery power. The module can even maintain accurate time if the battery power supply is interrupted.
- There is a built-in circuit which continuously senses power and if the battery supply is cut, then it automatically starts the backup power supply. So even during power outages, the module still keeps track of accurate time.
- This module uses 20mm 3V coin cells.
- DS3231 chip also has a 32 bytes EEPROM chip from Atmel with unlimited Read/writes. So it can be used to save anything.
- This chip also uses I2C communication protocol for interfacing. So both DS3231 chip and EEPROM chip share the same I2C bus for data transfer.
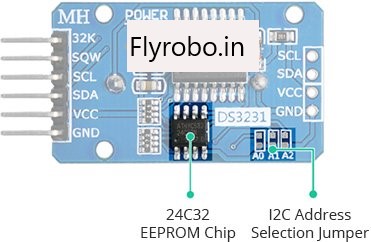
- The I2C address can be changed easily using the three I2C address jumper pins provided on the board.
- The I2C address jumper pins consist of the last three pins of a 7-bit I2C address just before the Read/Write bit. As there are three variables each with two different outputs, so there are total 8 combinations achievable.

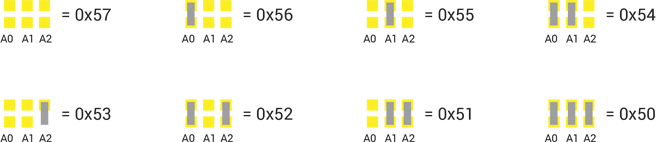
- By default, all the pins are pulled HIGH so the address by default is 0x57.
Pinout of the DS3231 module
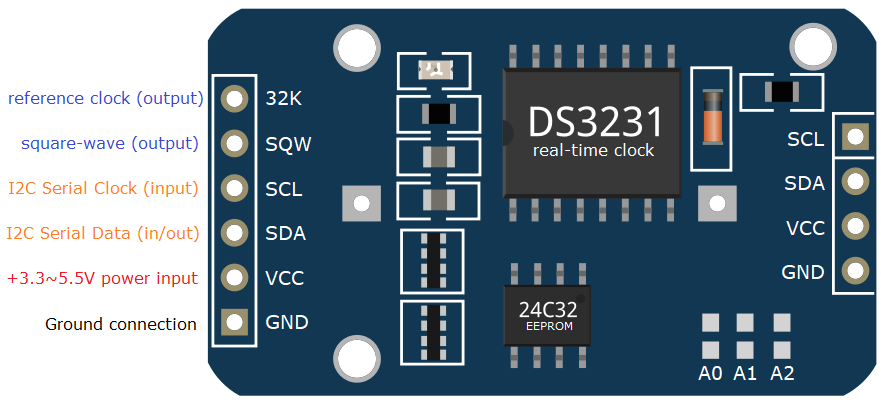
- The 32K pin outputs an accurate reference clock.
- The SQW pin outputs a square wave. These waves can have the frequency of 1kHz, 4kHZ, 8kHZ, 32kHZ and can be switched programmatically.
- The SCL is the serial clock pin for I2C interface.
- The SDA is the serial data pin for I2C interface.
- The VCC pin is the power input pin.
- The GND is the ground pin.
Circuit Diagram
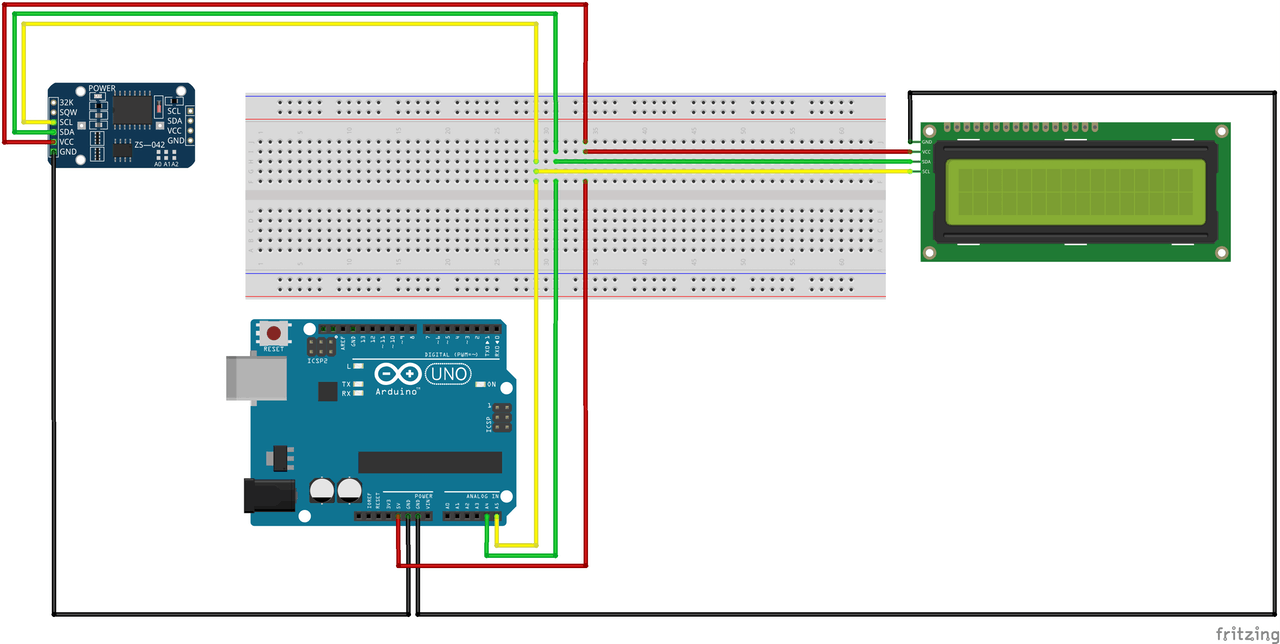
- Both the DS3231 and LCD work on the same I2C interface
- Both the SCL pins of the DS3231 and the LCD are connected to Arduino analog pin A5.
- Both the SDA pins of the DS3231 and the LCD are connected to Arduino analog pin A4.
- Both VCC and GND pins of DS3231 module and LCD are connected to 5V and GND pins respectively.
Working concept
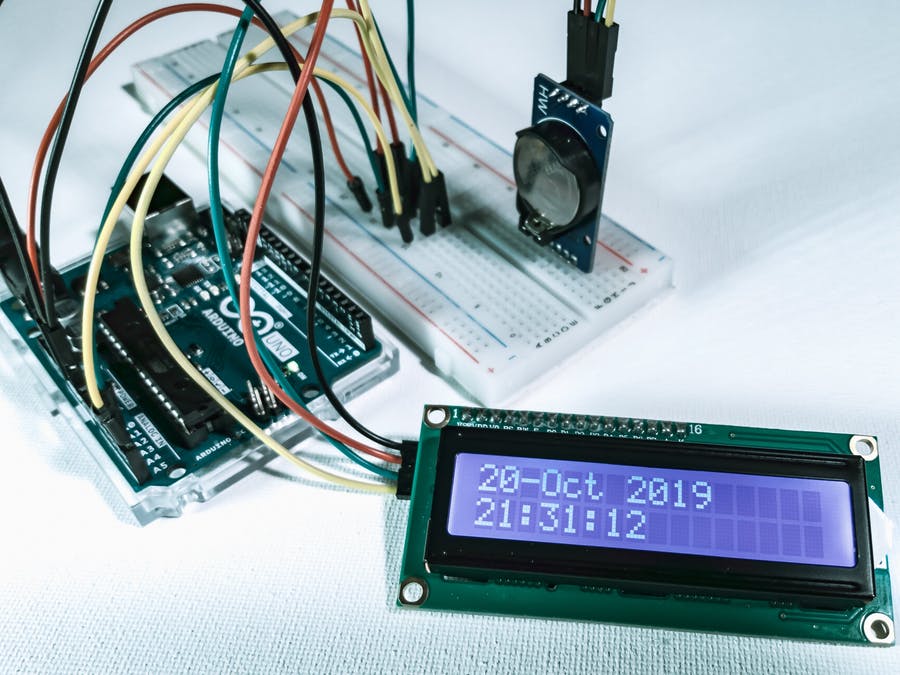
- The working concept of this project is pretty rudimentary.
- The DS3231 keeps track of accurate date and time. It sends this data to the Arduino board.
- The Arduino then converts this data into a compatible format for the LCD Display.
- Then Arduino sends this data to the LCD Display to show to the user.
Arduino Code
#define VISUINO_ARDUINO_UNO #include <OpenWire.h> #include <Mitov.h> #include <Wire.h> #include <Mitov_Basic_I2C.h> #include <Mitov_LiquidCrystalDisplay_I2C.h> #include <Mitov_RTC_DS1307.h> #include <Mitov_DateTime.h> #include <Mitov_ValueArray.h> namespace ComponentVariables { class { public: uint32_t Value1 : 5; uint32_t Value2 : 2; } BitFields; class Variable1 { public: inline static uint32_t GetValue() { return BitFields.Value1; } inline static void SetValue( uint32_t AValue ) { BitFields.Value1 = AValue; } }; class Variable2 { public: inline static uint32_t GetValue() { return BitFields.Value2; } inline static void SetValue( uint32_t AValue ) { BitFields.Value2 = AValue; } }; } namespace VisuinoConstants { class TextValue1 { public: inline static constexpr const char *GetValue() { return ""; } }; class TextValue2 { public: inline static constexpr const char *GetValue() { return " : : "; } }; class TextValue0 { public: inline static constexpr const char *GetValue() { return " - -"; } }; } namespace PinCalls { class PinCallerReceive0 { public: void Notify( void *_Data ); }; class PinCallerReceive1 { public: void Notify( void *_Data ); }; class PinCallerReceive2 { public: void Notify( void *_Data ); }; class PinCallerReceive3 { public: void Notify( void *_Data ); }; class PinCallerReceive4 { public: void Notify( void *_Data ); }; class PinCallerReceive5 { public: void Notify( void *_Data ); }; class PinCallerReceive6 { public: void Notify( void *_Data ); }; class PinCallerReceive7 { public: void Notify( void *_Data ); }; } // PinCalls // Declarations namespace Declarations { Mitov::LiquidCrystalDisplay< Mitov::LiquidCrystalDisplayI2C< TwoWire, // 0_TYPE_PIN Wire, // 1_NAME_PIN 39, // Address Mitov::ConstantProperty<14, bool, false >, // AutoScroll Mitov::ConstantProperty<4, bool, true >, // Backlight Mitov::ConstantProperty<3, bool, true >, // BacklightPositive Mitov::ConstantProperty<17, bool, false >, // Blink Mitov::ConstantProperty<13, bool, true >, // Enabled Mitov::ConstantProperty<18, bool, false >, // Hight10Pixels Mitov::ConstantProperty<15, bool, false >, // RightToLeft Mitov::ConstantProperty<16, bool, false > // ShowCursor >, // 0_TYPE 16, // Columns Mitov::TypedVariable<32, uint32_t, ::ComponentVariables::Variable2>, // FCursorLine Mitov::TypedVariable<32, uint32_t, ::ComponentVariables::Variable1>, // FCursorPos 2 // Rows > _o_LiquidCrystalDisplay1; Mitov::LiquidCrystalElementTextField< Mitov::LiquidCrystalDisplay< Mitov::LiquidCrystalDisplayI2C< TwoWire, // 0_TYPE_PIN Wire, // 1_NAME_PIN 39, // Address Mitov::ConstantProperty<14, bool, false >, // AutoScroll Mitov::ConstantProperty<4, bool, true >, // Backlight Mitov::ConstantProperty<3, bool, true >, // BacklightPositive Mitov::ConstantProperty<17, bool, false >, // Blink Mitov::ConstantProperty<13, bool, true >, // Enabled Mitov::ConstantProperty<18, bool, false >, // Hight10Pixels Mitov::ConstantProperty<15, bool, false >, // RightToLeft Mitov::ConstantProperty<16, bool, false > // ShowCursor >, // 0_TYPE 16, // Columns Mitov::TypedVariable<32, uint32_t, ::ComponentVariables::Variable2>, // FCursorLine Mitov::TypedVariable<32, uint32_t, ::ComponentVariables::Variable1>, // FCursorPos 2 // Rows >, // 0_TYPE_OWNER Declarations::_o_LiquidCrystalDisplay1, // 1_NAME_OWNER Mitov::ConstantProperty<5, bool, true >, // AllignLeft Mitov::ConstantProperty<7, uint32_t, 0 >, // Column Mitov::ConstantProperty<6, char, ' ' >, // FillCharacter Mitov::ConstantPropertyString<3, ::VisuinoConstants::TextValue0 >, // InitialValue Mitov::ConstantProperty<8, uint32_t, 0 >, // Row Mitov::ConstantProperty<4, uint32_t, 16 > // Width > TArduinoLiquidCrystalElementTextField1; Mitov::LiquidCrystalElementIntegerField< Mitov::LiquidCrystalDisplay< Mitov::LiquidCrystalDisplayI2C< TwoWire, // 0_TYPE_PIN Wire, // 1_NAME_PIN 39, // Address Mitov::
Leave a Comment