16 Aug
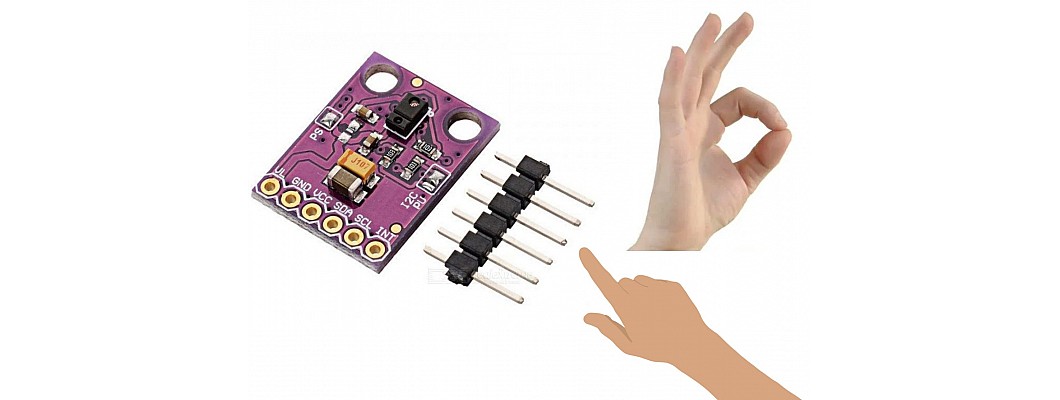
Hello,
In this article, we are going to learn about the gesture sensor APDS-9960.
Hardware Required
Software Required
- A gesture is a way of non-verbal communication where the person tries to send a message without words. These gestures can be performed using the movement of hands.
APDS-9960 Gesture Sensor
- The APDS-9960 gesture sensor has many functions. It is an infrared sensor. It can detect ambient light, gestures of any sort, and also RGB values in the light. The operating voltage of this sensor is 3.3V. It uses an I2C communication protocol for interfacing with Arduino.
- The sensor has an IR LED and 4 photodiodes. The LED emits IR waves and the photodiodes detect the reflected IR waves. The photodiodes are also constantly looking for IR radiation which can be emitted by objects.
- This received energy by the photodiodes is processed further and sent to the microcontroller board.
Pinout of APDS-9960
Pins | Description |
VL |
Optional Power to the IR LED |
GND | Ground Pin |
VCC | Used as the main power supply pin for the Sensor |
SDA | I2C Data pin |
SCL | I2C Clock pin |
INT | External interrupt pin |
Interfacing with Arduino
- For interfacing, we will be using a servo motor with Arduino which will rotate in the direction of the hand movement.
Pins on gesture sensor |
Pins on Arduino Uno |
VCC |
3.3V |
GND |
GND |
SCL |
A5 |
SDA |
A4 |
INT |
Digital pin 2 |
Pins on Servo motor |
Pins on Arduino Uno |
VCC |
5V |
GND |
GND |
Signal pin |
Digital Pin 9 |
Arduino Code
#include <Wire.h>
#include <Servo.h>
#include <SparkFun_APDS9960.h>
Servo myservo;
#define APDS9960_INT 2
SparkFun_APDS9960 apds = SparkFun_APDS9960();
int isr_flag = 0;
void setup() {
myservo.attach(9);
pinMode(APDS9960_INT, INPUT);
Serial.begin(9600);
Serial.println();
Serial.println(F("--------------------------------"));
Serial.println(F("SparkFun APDS-9960 - GestureTest"));
Serial.println(F("--------------------------------"));
attachInterrupt(0, interruptRoutine, FALLING);
if ( apds.init() ) {
Serial.println(F("APDS-9960 initialization complete"));
} else {
Serial.println(F("Something went wrong during APDS-9960 init!"));
}
if ( apds.enableGestureSensor(true) ) {
Serial.println(F("Gesture sensor is now running"));
} else {
Serial.println(F("Something went wrong during gesture sensor init!"));
}
}
void loop() {
if( isr_flag == 1 ) {
detachInterrupt(0);
handleGesture();
isr_flag = 0;
attachInterrupt(0, interruptRoutine, FALLING);
}
}
void interruptRoutine() {
isr_flag = 1;
}
void handleGesture() {
if ( apds.isGestureAvailable() ) {
switch ( apds.readGesture() ) {
case DIR_UP:
Serial.println("UP");
break;
case DIR_DOWN:
Serial.println("DOWN");
break;
case DIR_LEFT:
Serial.println("LEFT");
myservo.write(180); // added by RoboJax
break;
case DIR_RIGHT:
Serial.println("RIGHT");
myservo.write(0); // added by RoboJax
break;
case DIR_NEAR:
Serial.println("NEAR");
break;
case DIR_FAR:
Serial.println("FAR");
break;
default:
Serial.println("NONE");
}
}
}
- Upload the code to the Arduino board. Perform the circuit diagram.
- Now as you move your hand in front of the sensor, the servo motor will rotate in the same direction as that of the movement of the hand.
- That is all. I hope you learned something about the gesture sensor in this article. And I hope you liked it. Thank you.
Leave a Comment